How to send weekly emails to Google Workspace users without their profile photo uploaded.
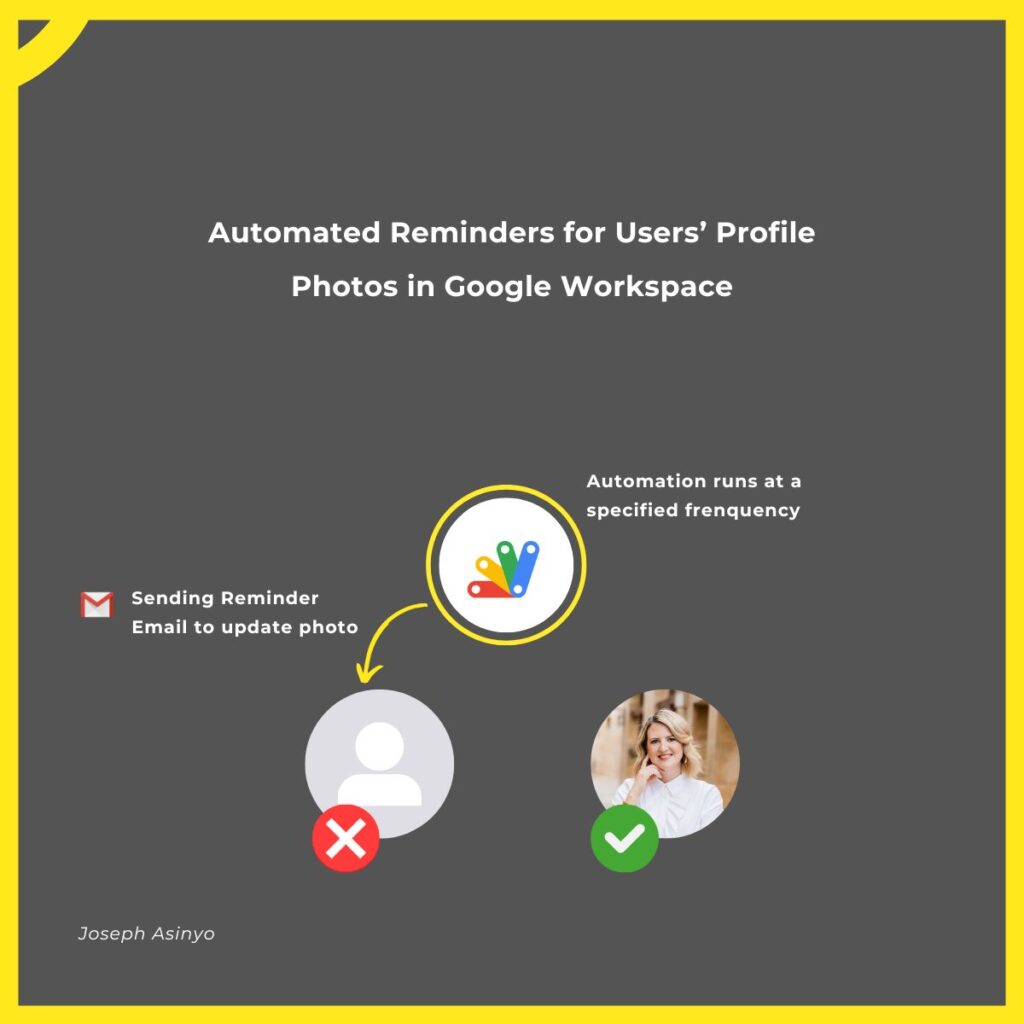
Do you want to encourage all users in your Google Workspace to set their profile photos for a more professional appearance? You don’t want to manually send them emails.
Try my simple automation instead. It runs at a set frequency to identify users without profile photos and sends them an email reminder. Follow-up reminders will be sent at the same frequency until users update their photos.
Benefit of the automation
Firstly, this automation encourages users to complete their profiles, making them easier to identify and interactions more friendly. Secondly, it removes the need for manual checks, saving administrators time for other important tasks.
How to set up the automation
You need to do some configuration to activate the automation as shown in the GIF below:
1. Make a copy of this spreadsheet
2. Grant Google permissions
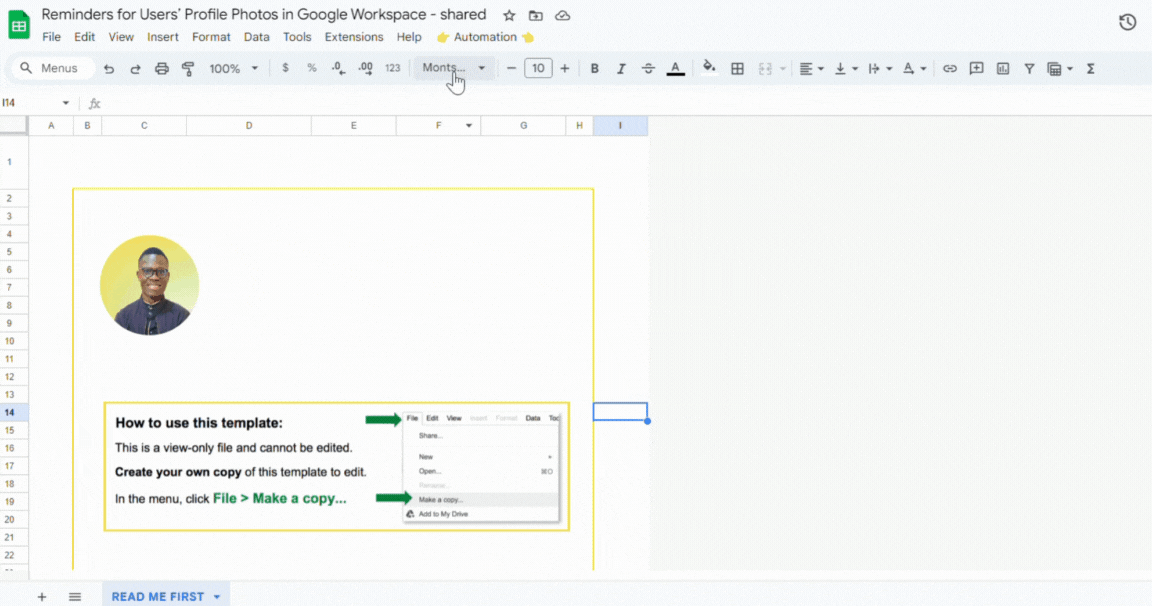
3. Create a trigger that will run the automation every day
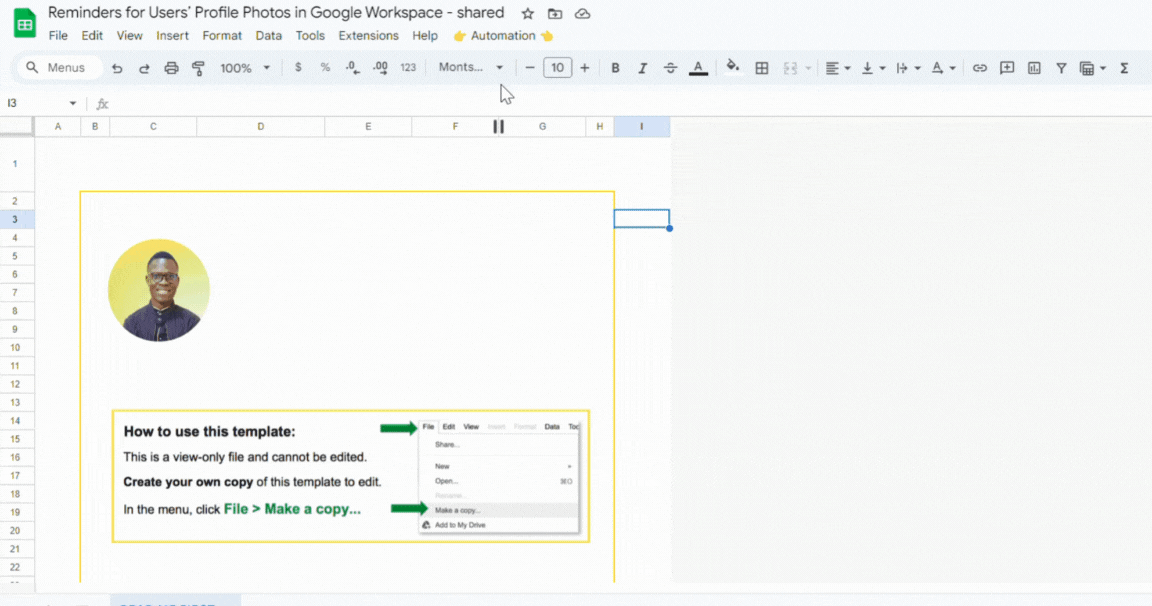
Script and explanation
This script uses an advanced Google service named AdminDirectory. So it has been added to the project via the Google Script editor. We will use 2 methods of this service to build our automation:
AdminDirectory.Users.list()
:
We will first use this method to fetch all the users in the Google Workspace.AdminDirectory.Users.Photos.get()
:
Then, for each user, we will use this method to check if they have a profile photo.
Finally, when the profile photo is not found for a user, we send them a reminder email using the MailApp.sendEmail()
method.
Here is the whole script:
/**
* Reminders for Users’ Profile Photos in Google Workspace
*
* Written with ❤️ by Joseph Asinyo
*/
function checkUserProfilePhotos(){
const users = AdminDirectory.Users.list().users;
users.forEach(user => {
try {
const userPhoto = AdminDirectory.Users.Photos.get(user.primaryEmail);
} catch (error) {
// user does not have a profile photo
// send reminder email
MailApp.sendEmail({
to: user.primaryEmail,
subject: "Please upload your profile photo",
body: "It looks like you haven't uploaded a profile photo. Please upload one."
});
}
});
}
function onOpen() {
var ui = SpreadsheetApp.getUi();
// Add a custom menu to the spreadsheet.
ui.createMenu('👉 Automation 👈')
.addItem('Grant Google Permissions', 'grantGooglePermissions')
.addSubMenu(ui.createMenu('Create Reminder Trigger')
.addItem('Create Trigger', 'createReminderTrigger')
.addItem('Delete Trigger', 'deleteReminderTrigger'))
.addToUi();
}
function grantGooglePermissions() {
// Function to grant Google Permissions.
FormApp
// You can add the necessary code to request permissions here.
SpreadsheetApp.getUi().alert('Google Permissions granted.');
}
function createReminderTrigger() {
var ui = SpreadsheetApp.getUi();
var response = ui.prompt('Create Reminder Trigger', 'Enter the number of days:', ui.ButtonSet.OK_CANCEL);
if (response.getSelectedButton() == ui.Button.OK) {
var days = parseInt(response.getResponseText(), 10);
if (isNaN(days) || days <= 0) {
ui.alert('Please enter a valid number of days.');
return;
}
// Check if the trigger already exists
var triggers = ScriptApp.getProjectTriggers();
var triggerExists = triggers.some(trigger => trigger.getHandlerFunction() === 'checkUserProfilePhotos');
if (triggerExists) {
ui.alert('A trigger for this function already exists.');
} else {
// Create the new trigger
ScriptApp.newTrigger('checkUserProfilePhotos')
.timeBased()
.everyDays(days)
.create();
ui.alert('Trigger created to run every ' + days + ' days.');
}
}
}
function deleteReminderTrigger() {
var ui = SpreadsheetApp.getUi();
var triggers = ScriptApp.getProjectTriggers();
var triggerExists = false;
triggers.forEach(function(trigger) {
if (trigger.getHandlerFunction() === 'checkUserProfilePhotos') {
ScriptApp.deleteTrigger(trigger);
triggerExists = true;
}
});
if (triggerExists) {
ui.alert('Trigger deleted successfully.');
} else {
ui.alert('No trigger found.');
}
}