In this post, you will learn how to build a custom function that imports into a sheet the details of YouTube channels given their names.
Here is how the function works:
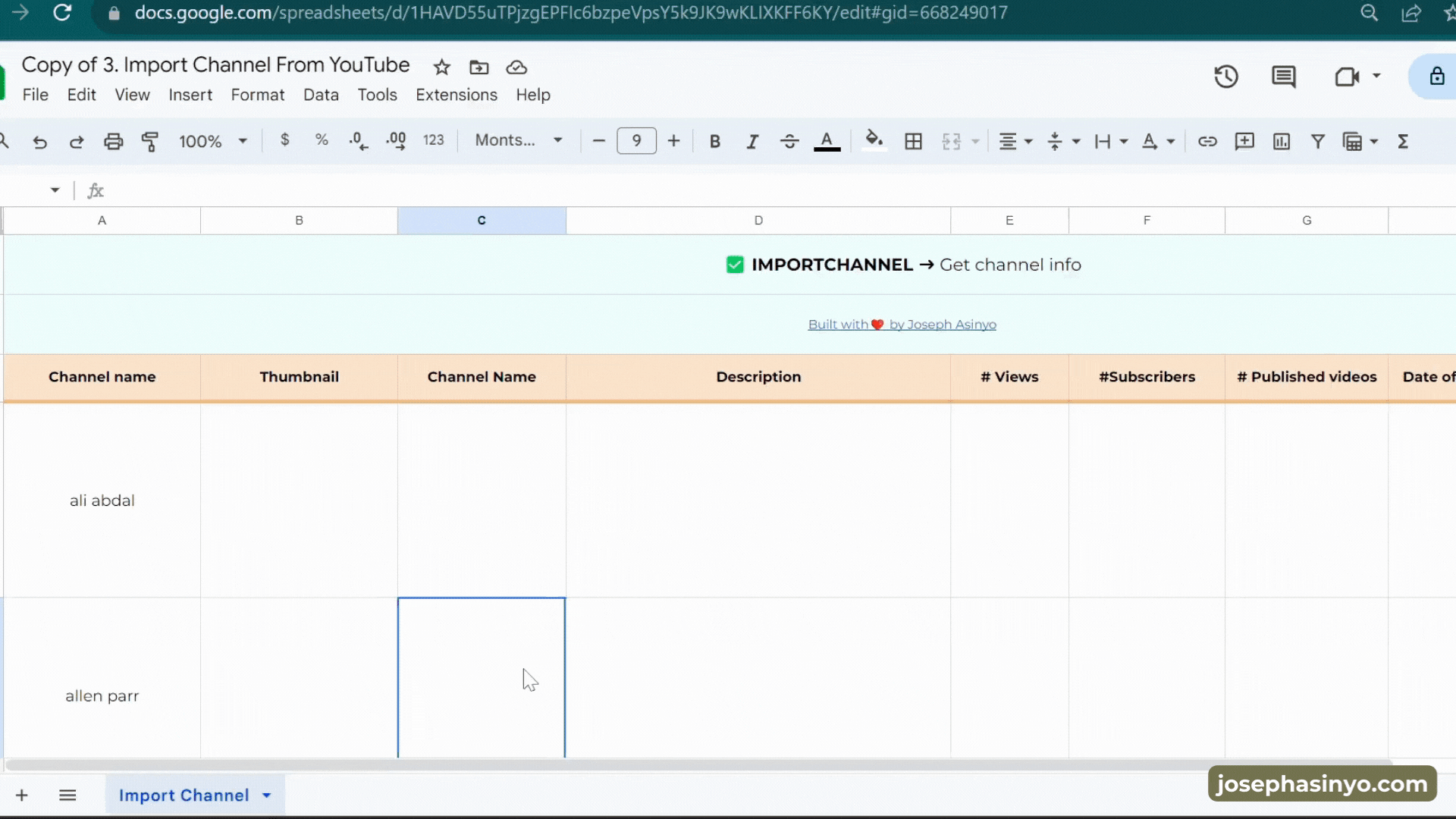
This feature can be useful for tracking channel performance and conducting research.
1. Get the template
Click the button below to copy the template sheet with the custom function ready for use. Once you get your copy, all you need to do is follow the short video tutorial to initialize the script.
In the following lines, I’ll explain how I developed this function. So, stick with me if you’re interested in more details.
2. Creating the core function
The first thing we need to do is enable the YouTube Data API. This advanced service provides us with broad access to YouTube’s public data. It allows us to fetch details about videos. To enable it, open your Google Script editor, then go to Resources > Advanced Google Services > YouTube Data API > Enable.
Next, let’s create a core function that takes a YouTube channel name as input and retrieves the details about the channel, including the channel’s name, description, number of views, number of subscribers, the total number of published videos, the date of channel creation, the thumbnail URL, and the link to the channel.
function getChannelData(channelName) {
// Fetch the channel's ID
var searchResponse = YouTube.Search.list('snippet', {
q: channelName,
type: 'channel',
maxResults: 1
});
// Check if the channel exists
if (searchResponse.items.length === 0) {
return "Channel not found";
}
// Get the channel's ID
var channelId = searchResponse.items[0].id.channelId;
// Fetch the channel information
var channelInfo = YouTube.Channels.list('snippet,statistics', {
id: channelId
});
var publishDate = new Date(channelInfo.items[0].snippet.publishedAt);
var formattedDate = publishDate.toLocaleDateString("en-US", { month: "2-digit", day: "2-digit", year: "numeric" });
var formattedTime = publishDate.toLocaleTimeString("en-US", { hour: "2-digit", minute: "2-digit" });
var formattedDateTime = formattedDate + " " + formattedTime;
// Extract desired information from the response
var channelDetails = {
title: channelInfo.items[0].snippet.title,
description: channelInfo.items[0].snippet.description,
viewCount: parseInt(channelInfo.items[0].statistics.viewCount),
subscriberCount: parseInt(channelInfo.items[0].statistics.subscriberCount),
videoCount: parseInt(channelInfo.items[0].statistics.videoCount),
publishAt: formattedDateTime,
thumbnail: channelInfo.items[0].snippet.thumbnails.high.url
};
var result = {data: [[
channelDetails.title,
channelDetails.description,
channelDetails.viewCount,
channelDetails.subscriberCount,
channelDetails.videoCount,
channelDetails.publishAt,
channelDetails.thumbnail
]]};
// Return the channel information as an array
console.log(result);
return result;
}
3. Creating the custom function
If we try to call getChannelData(), we will get an error indicating that the call to the YouTube Data API has failed. The reason is that custom functions in Google Sheets run with limited authorization and are unable to call advanced services that require user authorization, such as the YouTube Data API.
So we need to find a way to grant our custom function the necessary permissions to run. As a solution, we will first deploy the script as a web app, which will grant the necessary permissions for the function to access the YouTube Data API. Then, we’ll use the URL of the web app to make HTTPS requests to the core function getChannelData().
For this reason, we need to add a few functions to the code:
- IMPORTCHANNEL(videoLink):
We will create a wrapper function IMPORTCHANNEL that will finally become the actual custom function. Whenever this custom function is called from the sheet, instead of trying to call the core function directly (which would fail due to insufficient permissions), a request will be made to the web app we deployed. The web app, with its sufficient permissions, will then call the getChannelData() function and return the results. This way, we can successfully access the YouTube Data API. - makeRequestToWebApp(parameters):
This function is responsible for making a request to the web app. It uses the UrlFetchApp.fetch method to make the HTTP request. The response from the web app is then parsed and returned. - doGet(e)
This function is automatically executed when a GET request is made to the URL of the web app. In this script, thedoGet
function checks the request_type parameter of the request. If the request_type is “importchannel”, it calls the getChannelData() function with the videoLink parameter from the request. The result of thegetVideoData
function is then turned into a string and returned.
Here is the updated script:
/**
* Fetches channel information from YouTube API based on a channel name.
*
* @param {string} channelName The name of the YouTube channel.
* @return {Object} The channel information.
* @customfunction
*/
function IMPORTCHANNEL(channelName) {
var parameters = {
"request_type": "importchannel",
"channelName": channelName,
}
var result = makeRequestToWebApp(parameters)
return result;
}
function makeRequestToWebApp(parameters) {
var url = WEB_APP_URL+"?";
for (const key of Object.keys(parameters)) {
url += key+"="+parameters[key]+"&";
}
url = url.slice(0, -1); // Remove the last "&" character
console.log(url)
var response = UrlFetchApp.fetch(url).getContentText();
var result = JSON.parse(response).data;
return result;
}
// Web Apps using as the wrapper.
function doGet(e) {
var request_type = e.parameter.request_type
if(request_type == "importchannel"){
var res = getChannelData(e.parameter.channelName)
}
return ContentService.createTextOutput(JSON.stringify(res));
}
function getChannelData(channelName) {
// Fetch the channel's ID
var searchResponse = YouTube.Search.list('snippet', {
q: channelName,
type: 'channel',
maxResults: 1
});
// Check if the channel exists
if (searchResponse.items.length === 0) {
return "Channel not found";
}
// Get the channel's ID
var channelId = searchResponse.items[0].id.channelId;
// Fetch the channel information
var channelInfo = YouTube.Channels.list('snippet,statistics', {
id: channelId
});
var publishDate = new Date(channelInfo.items[0].snippet.publishedAt);
var formattedDate = publishDate.toLocaleDateString("en-US", { month: "2-digit", day: "2-digit", year: "numeric" });
var formattedTime = publishDate.toLocaleTimeString("en-US", { hour: "2-digit", minute: "2-digit" });
var formattedDateTime = formattedDate + " " + formattedTime;
// Extract desired information from the response
var channelDetails = {
title: channelInfo.items[0].snippet.title,
description: channelInfo.items[0].snippet.description,
viewCount: parseInt(channelInfo.items[0].statistics.viewCount),
subscriberCount: parseInt(channelInfo.items[0].statistics.subscriberCount),
videoCount: parseInt(channelInfo.items[0].statistics.videoCount),
publishAt: formattedDateTime,
thumbnail: channelInfo.items[0].snippet.thumbnails.high.url
};
var result = {data: [[
channelDetails.title,
channelDetails.description,
channelDetails.viewCount,
channelDetails.subscriberCount,
channelDetails.videoCount,
channelDetails.publishAt,
channelDetails.thumbnail
]]};
// Return the channel information as an array
return result;
}
3. Usage capacity of the custom function
The number of times the getChannelData function can be used within a day depends on the quota limits set by the YouTube Data API. The default quota limit for the YouTube Data API is 10,000 units per day.
The getChannelData function makes two API calls: one to the Search.list method to find the channel and another to the Channels.list method to get the channel’s data. Each one of these methods costs 1 unit, so each call of the custom function will cost 2 units.
This means that you can call the IMPORTCHANNEL
function 5,000 times within a day (10,000 units divided by 2 units per function call).
The daily quota resets at midnight Pacific Time. If you reach the quota limit, you can request additional quota by completing the Quota extension request form for YouTube API Services.
Useful links: